Python - Command Line Argument
If you are at early stage of learning computer programming you may not see such a strong necessity of this kind of function, but as your programming experience gets longer and your skill gets better you would like to your script more flexible and let it work differently based on parameters that user specifies. This is where you see the necessity of Command Line Argument processing.
Command-line arguments are a way to pass input values to your script from the command line when executing it. These arguments can be used to customize the behavior of your script or provide data without having to hard-code it into the script itself. This makes your script more flexible and reusable.
Using argv Array
To work with command-line arguments in Python, you can use the sys.argv list from the sys module. The sys.argv list contains the command-line arguments that were passed to the script. The first item in the list, sys.argv[0], is the name of the script itself. The following items, sys.argv[1], sys.argv[2], and so on, correspond to the arguments provided when the script is called.
Here is an overview of the steps to work with command-line arguments in Python:
- Import the sys module: To access the sys.argv list, you need to first import the sys module using the statement import sys.
- Access the command-line arguments: You can access the command-line arguments using the sys.argv list. For example, to access the first argument, you can use sys.argv[1]. Keep in mind that the list is indexed starting from 0, and sys.argv[0] is the name of the script itself.
- Handle the arguments: You can process the command-line arguments within your script to customize its behavior based on the input values. This may include parsing the arguments, validating them, or using them as parameters for functions or other parts of your script.
- Handle exceptions: When working with command-line arguments, it's essential to handle potential exceptions that may occur due to missing or invalid input values. For example, you can use a try-except block to catch the IndexError exception that may be raised if the required arguments are not provided.
The sys.argv list is a simple way to access command line arguments. It is part of the built-in sys module and contains the list of command line arguments passed to a script. The first item in the list, sys.argv[0], is the name of the script itself. The following items are the arguments provided by the user.
Example 01 >
Create a file named CommandLineArgTest.py and type in following code
CommandLineArgTest.py |
import sys
print("The name of the script file: ", sys.argv[0])
print("Number of the command line arguments: ", len(sys.argv))
print("The arguments are: " , str(sys.argv))
argList = ["apple","banana","strawberry"]
i = 0;
for cmd in sys.argv :
print("Argument[", i, "] = ",cmd)
i = i + 1
|
You can run the script with the command line argument as shown below.
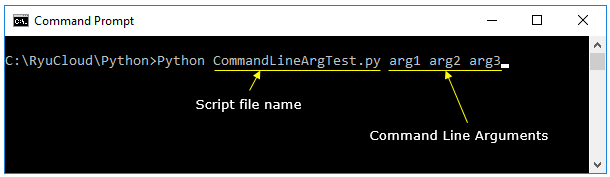
Then you should have the result as shown below.
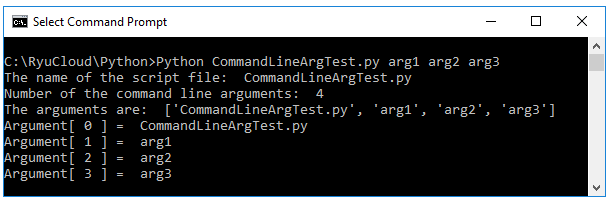
With the same script, you can pass any number of arguments as shown below (i put different number of space between arguments to show that the number of space between arguments does not matter)
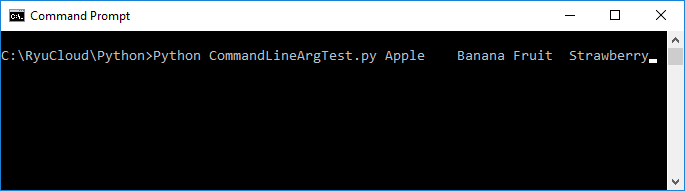
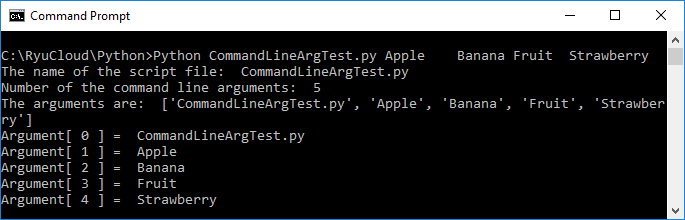
Using argparse Module
argparse is a powerful library for creating user-friendly command-line interfaces. It allows you to easily parse command-line arguments, provide help messages, and display error messages. With argparse, you can define the arguments your script accepts, specify their types, and even set default values or make them required.
Here's an overview of the key concepts and steps to work with argparse in Python:
- Import the argparse module: To use argparse, you need to first import the module using the statement import argparse.
- Create an ArgumentParser object: To start working with command-line arguments, you need to create an instance of the argparse.ArgumentParser class. This object will handle parsing the command-line arguments and generating help messages.
- Add arguments to the parser: You can add arguments to the ArgumentParser object using the add_argument() method. This method allows you to specify the name of the argument, its type, default value, whether it's required or optional, and a help message. You can add positional arguments, which are mandatory and must be provided in a specific order, as well as optional arguments, which are preceded by a flag (e.g., -f or --file).
- Parse the command-line arguments: Once you have defined all the arguments your script accepts, you can parse the command-line input using the parse_args() method of the ArgumentParser object. This method returns an object containing the values of the provided arguments, which can be accessed as attributes of the object.
- Use the parsed arguments: After parsing the command-line arguments, you can use the values in your script to customize its behavior based on the input. You can access the values of the arguments using the attributes of the object returned by parse_args().
- Handle errors and display help messages: One of the main advantages of using argparse is its built-in error handling and help message generation. If the user provides invalid input or requests help using the -h or --help flag, argparse will automatically generate an appropriate message and display it to the user.
The argparse module provides a more powerful and user-friendly way to handle command line arguments. It allows you to define the arguments that your script accepts, specify their types, and provide help messages. The argparse.ArgumentParser class is used to create a parser that processes command line arguments.
Example 01 > all the argement in a single array
Create a file named CommandLineArgParser_01.py and type in following code In this example, all the command line argument is stored in a single array variable named args.
CommandLineArgParser_01.py |
import argparse
# Create the argument parser
parser = argparse.ArgumentParser(description="A script to demonstrate argparse usage")
# Add arguments to the parser
parser.add_argument("args", metavar="arg", type=str, nargs="*", help="a list of arguments")
# Parse the command line arguments
args, unknown = parser.parse_known_args()
# Print information about the script and its arguments
print("The name of the script file:", parser.prog)
print("Number of the command line arguments:", len(args.args) + 1)
print("The arguments are:", [parser.prog] + args.args)
# Print the parsed arguments
print("Command Line Arguments:")
for i, arg in enumerate(args.args):
print(f"Argument[{i + 1}] =", arg)
|
Command Prompt
|
C:\> python CommandLineArgParser_01.py apple banana strawberry
|
Command Prompt
|
C:\> python CommandLineArgParser_01.py apple banana strawberry
The name of the script file: CommandLineArgParser_01.py
Number of the command line arguments: 4
The arguments are: ['CommandLineArgParser_01.py', 'apple', 'banana', 'strawberry']
Command Line Arguments:
Argument[1] = apple
Argument[2] = banana
Argument[3] = strawberry
|
With the same script, you can pass any number of arguments as shown below (i put different number of space between arguments to show that the number of space between arguments does not matter)
Command Prompt
|
C:\> python CommandLineArgParser_01.py apple banana strawberry
|
Command Prompt
|
C:\> python CommandLineArgParser_01.py apple banana strawberry
The name of the script file: CommandLineArgParser_01.py
Number of the command line arguments: 4
The arguments are: ['CommandLineArgParser_01.py', 'apple', 'banana', 'strawberry']
Command Line Arguments:
Argument[1] = apple
Argument[2] = banana
Argument[3] = strawberry
|
Example 02 > each of the arguments stored in separate parameters
Create a file named CommandLineArgParser_02.py and type in following code In this example, each of the command line arguments is stored in separate variables..
CommandLineArgParser_02.py |
import argparse
# Create the argument parser
parser = argparse.ArgumentParser(description="A script that takes exactly three arguments")
# Add arguments to the parser
parser.add_argument("arg1", help="First argument")
parser.add_argument("arg2", help="Second argument")
parser.add_argument("arg3", help="Third argument")
# Parse the command line arguments
args = parser.parse_args()
# Access the parsed arguments and print them
print("arg1:", args.arg1)
print("arg2:", args.arg2)
print("arg3:", args.arg3)
|
Command Prompt
|
C:\> python CommandLineArgParser_02.py apple banana strawberry
|
Command Prompt
|
C:\> python CommandLineArgParser_02.py apple banana strawberry
arg1: apple
arg2: banana
arg3: strawberry
|
Example 03 > split the arguments into multiple groups
Create a file named CommandLineArgParser_03.py and type in following code In this example, the arguments are grouped into multiple groups. The first arguments is stored to arg1, the second and third arguments are stored to arg2, fourth and fifth arguments are stored to arg3.
CommandLineArgParser_03.py |
import argparse
# Create the argument parser
parser = argparse.ArgumentParser(description="A script that takes 5 arguments")
# Add arguments to the parser
parser.add_argument("arg1", help="First argument")
parser.add_argument("arg2", nargs=2, help="Second and third arguments")
parser.add_argument("arg3", nargs=2, help="Fourth and fifth arguments")
# Parse the command line arguments
args = parser.parse_args()
# Access the parsed arguments and print them
print("arg1:", args.arg1)
print("arg2:", args.arg2)
print("arg3:", args.arg3)
|
Command Prompt
|
C:\> python CommandLineArgParser_03.py apple banana strawberry pineapple blueberry
|
Command Prompt
|
C:\> python CommandLineArgParser_03.py apple banana strawberry pineapple blueberry
arg1: apple
arg2: ['banana', 'strawberry']
arg3: ['pineapple', 'blueberry']
|
Example 04 > error handling
Create a file named CommandLineArgParser_04.py and type in following code In this example, I will show you how to handle errors when you have a missing argument.
CommandLineArgParser_04.py |
import argparse
import sys
# Create the argument parser
parser = argparse.ArgumentParser(description="A script that takes 5 arguments")
# Add arguments to the parser
parser.add_argument("arg1", help="First argument")
parser.add_argument("arg2", nargs=2, help="Second and third arguments")
parser.add_argument("arg3", nargs=2, help="Fourth and fifth arguments")
try:
# Parse the command line arguments
args = parser.parse_args()
except SystemExit:
print("Error: Missing required arguments")
sys.exit(1)
# Access the parsed arguments and print them
print("arg1:", args.arg1)
print("arg2:", args.arg2)
print("arg3:", args.arg3)
|
Following sample run shows how to print help
Command Prompt
|
C:\> python CommandLineArgParser_04.py -h
usage: CommandLineArgParser_04.py [-h] arg1 arg2 arg2 arg3 arg3
A script that takes 5 arguments
positional arguments:
arg1 First argument
arg2 Second and third arguments
arg3 Fourth and fifth arguments
optional arguments:
-h, --help show this help message and exit
Error: Missing required arguments
|
Following sample run shows how the error is handled when you have missing argument
Command Prompt
|
C:\> python CommandLineArgParser_04.py apple banana strawberry pineapple
usage: CommandLineArgParser_04.py [-h] arg1 arg2 arg2 arg3 arg3
CommandLineArgParser_04.py: error: the following arguments are required: arg3
Error: Missing required arguments
|
Following sample run shows the result of normal run
Command Prompt
|
C:\> python CommandLineArgParser_04.py apple banana strawberry pineapple blueberry
arg1: apple
arg2: ['banana', 'strawberry']
arg3: ['pineapple', 'blueberry']
|
|
|